Eigentlich wollte ich recherchieren, welche Sessions auf der Microsoft BUILD Konferenz präsentiert werden um einen Eindruck von den Themen zu bekommen, die Microsoft wichtig sind. Man kann sich dort durch die Sessions Blättern und es scheint eine SPA JavaScript Page zu sein. Beides völlig falsch für den Anwendungsfall. Die ng- Attribute im HTML Source lassen vermuten, das es sich um AngularJS, also der ersten Version handeln. Leicht schräg. Jedenfalls lässt sich so der API REST Service anzapfen und ein alternatives UI mit .. tatata ASP.NET Core Razor Pages bauen und das natürlich gleich in der Version 3.
Das Projekt wird in folgende Blöcke geteilt
- Reverse Engineering des REST Services
- ASP.NET Core 3 Projekt und Änderungen
- HTTPClient
- JSON in core
Api Call mit Fiddler mitschneiden
Der einfachste Part ist per Brower Tools oder Fiddler den Aufruf des REST Services mitzuschneiden. Dieser besteht aus einem POST der Url https://api.mybuild.techcommunity.microsoft.com/api/session/search. Im Header sollte man mitgeben Content-Type: application/json;charset=utf-8. Die Queryparameters landen im Headers Bereich. Um nicht Blätter zu müssen wird der Rowcounter ItemsPerPage drastisch auf 500 raufgeschraubt.
{"itemsPerPage":500,"searchText":"*","searchPage":0,"sortOption":"None","searchFacets":{"facets":[],"personalizationFacets":[],"dateFacet":{"startDateTime":"2019-05-05T08:00:00-07:00","endDateTime":"2019-05-10T03:59:59+02:00"}},"recommendedItemIds":[],"favoritesIds":[]}
DOTNET Core 3
Um ehrlich zu sein, wir befinden uns erst im Preview 2 Stadium und die Änderungen zu 2.2 sind nicht so dramatisch. Auch mit Visual Studio 2019 muss erst die aktuelle Version per Download und Installer in Betrieb genommen werden. Die Templates und das UI haben sich allerdings erheblich geändert. Wählen Sie Webanwendung (im Bild unten angeschnitten) aus.
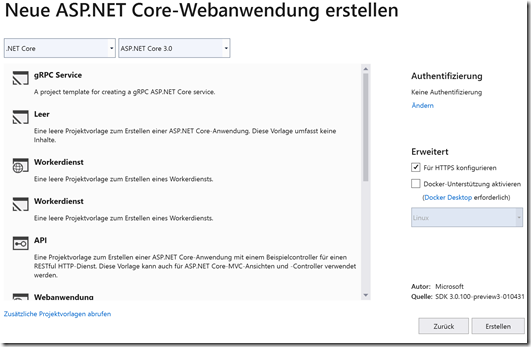
Ansonsten ist der Rest ident. Legen Sie eine Model Klasse an. Entweder Sie tippen per Hand die Properties oder lassen sich per Paste Special aus dem Json Daten in der Zwischenablage die Klassen generieren.
Microsoft behauptet das ASPNETCORE 30% schneller sei als die Vorgänger Version.
HTTPClient Client Factory
Was uns auch gleich zum nächsten Problem führt. Wenn alles so superschnell gehen soll, sind die Spassbremsen nicht förderlich. Ein REST Service Call vom Webserver auf einen anderen Server gehört defintiv in die Kategorie. Die Redmonder Entwickler haben aus diesem Grund eine optimierte Version des HTTP Clients im Angebot, der unter anderem Ressourcen schneller frei geben soll bzw so eine Art Connection Pooling betreibt. Um dies zu realisieren muss man verhindern´, beliebig viele Instanzen davon zu starten. Der Weg in ASP.NET Core führt über die Startup.cs und dem Befehl services.AddHttpClient();. Per Dependency Injection wird dann immer mit ein und derselben Instanz interagiert.
Für die Visualisierung wird eine Razor Page benötigt. Im Page Model bzw dem Konstuktor holt man sich die Referenz auf HttpClient per DI und sichert diese in einer internen Variablen.
1: public class SessionsPageModel : PageModel
2: {
3: private readonly IHttpClientFactory _clientFactory;
4:
5: public SessionsPageModel(IHttpClientFactory clientFactory)
6: {
7: _clientFactory = clientFactory;
8: }
Utf8JsonWriter statt JSON.NET
Der gute Mr Newton, seines Zeichen Erfinder der meistgenutzten JSON Libaray JSON.NET steht seit geraumer Zeit in Redmonder Diensten. Microsoft möchte, das wir den Nachfolger (mind 2,5 mal schneller) verwenden: Utf8JsonWriter oder auch Reader, je nachdem was man braucht. Erinnert irgendwie an alte SAX Parser. Man kann zwar JSON Zeichenketten in eine JsonDocument parsen, aber aktuell keinen vollständigen Type Cast wie man das mit JSON.NET deserializer mit einer Zeile Code (auch VB.NET) hinbekommt.
Der Weg ist steinig und man muss die Struktur des JSON Trees genau kennen.
Das komplette Code Beispiel. Das die Klasse Datum heißt ist ein dummer Zufall von Visual Studio. Hat mit Datum nichts zu tun,
1: public List<Datum> Sessions { get; set; } = new List<Datum>();
2: public async Task OnGetAsync()
3: {
4: //var client = new HttpClient();
5: var client = _clientFactory.CreateClient();
6: var content = new StringContent("{'itemsPerPage':500,'searchText':'*','searchPage':0,'sortOption':'None','searchFacets':{'facets':[],'personalizationFacets':[],'dateFacet':{'startDateTime':'2019-05-05T08:00:00-07:00','endDateTime':'2019-05-10T03:59:59+02:00'}},'recommendedItemIds':[],'favoritesIds':[]}");
7: content.Headers.ContentType = new MediaTypeHeaderValue("application/json");
8: var response = await client.PostAsync("https://api.mybuild.techcommunity.microsoft.com/api/session/search", content);
9: var json= response.Content.ReadAsStringAsync().GetAwaiter().GetResult();
10: using (JsonDocument doc = JsonDocument.Parse(json))
11: {
12: JsonElement root = doc.RootElement;
13: JsonElement info = root.GetProperty("data");
14: foreach (var item in info.EnumerateArray())
15: {
16: //deserializer???
17: Datum localItem = new Datum();
18: localItem.title = item.GetProperty("title").GetString();
19: localItem.description= item.GetProperty("description").GetString();
20: Sessions.Add(localItem);
21: }
22: }
und natürlich auch noch der aktuelle Stand der #MSBUILD Sessions
.NET Platform Overview and Roadmap
5 industries that are getting disrupted by Computer Vision on Cloud and on Edge
Accessibility and your development process: Making applications for everyone
Accessing data from anywhere with Excel custom functions and JavaScript API
Adaptive Cards vNext – Powering the next generation of contextual user experiences in your apps and Microsoft 365
AI in Power BI
All the Developer Things with Hanselman and Friends
Apply Knowledge Mining to your domain: Learn how to build custom skills that tailor content understanding to your specific industry
Architecting and Building Hybrid Cloud Apps for Azure and Azure Stack
Automate Caffeine delivery with a Teams 'CoffeeBot'
Automating background processes across Microsoft 365 data using Microsoft Graph and Azure
Azure AI: Powering AI for every developer and every organization
Azure IoT Edge + AI: Industry leading Edge computing accelerated with AI
Azure IoT platform services: a comprehensive overview
Azure Notebooks for Data Science Developers
Azure Security: Can you keep a secret?
Azure’s Data Platform - Powering Modern Applications and Cloud Scale Analytics at Petabyte Scale
Best practices for Azure Cosmos DB: Data modeling, Partitioning and RUs
Beyond the Brick and Mortar: How Wegmans delivers Innovation with API Architecture
Breaking the Wall between Data Scientists and App Developers with MLOps
Broadening implementation options of a .NET platform by adding a JavaScript SDK
Build an AI-powered Pet Detector with Python, TensorFlow, and Visual Studio Code
Build cloud native apps using MySQL that scale to 500 million transactions a day on Azure
Build large scale near real time analytical solutions to accelerate the digital feedback loop for IoT and Apps
Build Mission Critical .NET microservices
Build modern database applications using Azure SQL Database Managed Instance, ADF and Power BI
Building .NET Desktop Applications with .NET Core and Windows 10
Building a Microsoft Graph-powered productivity app
Building a new class of insight apps with bulk datasets from Microsoft Graph using Azure services
Building an App on the Power Platform
Building apps that integrate, automate, and manage security operations
Building Azure Apps using the Common Data Service
Building data connectors for the Microsoft Power Platform
Building data pipelines for Modern Data Warehouse with Spark and .NET in Azure
Building engaging, intelligent portals and solutions with SharePoint Framework
Building event driven apps with Azure Functions and Azure Cosmos DB change feed
Building mobile and web applications using .NET, macOS and Visual Studio for Mac
Building PostgreSQL apps at any scale with Hyperscale (Citus)
Building Progressive Web Apps to extend existing web apps in Windows and Microsoft Teams
Building Python Web Applications with Visual Studio Code, Docker, and Azure
Building the award winning app, SeeingAI, with Visual Studio 2019
Building thriving open source communities with GitHub
Built for Speed: SQL Server Database Application Design for Performance
Closing the key gaps of serverless with Azure Functions
Code-free data integration at scale for Azure SQL Data Warehouse with Azure Data Factory
Coding from scratch a realtime Azure data and AI pipeline
Cognitive Services for the Enterprises
Contributing and building extensions for Azure Data Studio
Creating Next Generation Business Apps with Dynamics, Power platform and Office
Creating your minimum viable product in under one (1) hour
Data Architect’s guide for successful Open Source patterns in Azure w/ Spark, Hive, Kafka, Hbase etc
Dataflows and data interop with the Common Data Model
Debugger/Diagnostics Tips & Tricks in Visual Studio 2019
Deep dive: Mission-critical multi-tenant apps with Cosmos DB multi-master
Deploying apps for on-premises and hybrid ML+AI on SQL Server 2019 big data clusters
Designing AI Responsibly
Designing for speech
Develop data application on a no-limits SQL data platform
Developing Business Applications via Microsoft Power Platform
Developing large-scale Azure HPC solutions with Batch and CycleCloud
Developing Mobile Augmented Reality (AR) Applications with Azure Spatial Anchors
Developing with Power BI embedded analytics
DevOps for applications running on Windows
Embed low-code/no-code capabilities in your app with PowerApps and Flow
Embedding analytics into multi-tenant SaaS Applications with Power BI Embedded: Best practices
Enabling your application and services to use passwordless authentication
End to end application development and DevOps on Azure Kubernetes Service
Enhance your existing application with cognitive services
Enterprise security in the era of containers and Kubernetes
Event-driven design patterns to enhance existing applications using Azure Functions
Fluent Design System: the journey to cross-platform
From cloud to the intelligent edge: Drive real-time decisions with Azure Stream Analytics
From dev to production: Container lifecycle, monitoring, logging and troubleshooting
From Monolith to Microservice: How Azure powered Vipps to become the No. 1 payment service in Norway
From Zero to AI Hero–Automatically generate ML models using Azure Machine Learning service, Automated ML
From Zero to DevOps Superhero: The Container Edition
Full stack web development with ASP.NET Core 3.0 and Blazor
Guide to migrate your .NET app to Azure
How to build enterprise ready ML: Privacy and Security best practices, in the cloud and on the edge
How to use Azure Conversational AI to scale your business for the next generation- A deep dive into La Liga’s story
How XBox delivered the Right Experiences & Content with Reinforcement Learning
Implementing control at scale in a cloud & devops centric environment using policy and blueprints
Increase your .NET Productivity with Visual Studio 2019
Infrastructure as Code (or Continuous Delivery Infrastructure)
Intro to Building Apps for HoloLens 2 Using Unity and Mixed Reality Toolkit
Intro to Building Mixed Reality Apps with Unreal
Intro to Developing for Azure Kinect
IoT Security from Sensors to Cloud
JavaScript for Artists
Lessons learned from building Candy Crush for Windows 10
Leveraging WSL For DevOps and Cross-Platform Development
Managing your ML lifecycle with Azure Databricks and Azure ML
Maximizing existing solutions and services in Microsoft Teams
Meet C++/WinRT 2.0: Faster, smarter, and in the open
Microsoft Flow & Graph API - Automated processing of information intelligently
Microsoft Graph: Powering your applications with Microsoft 365 services
Microsoft IoT Vision and Roadmap
Microsoft Power BI: the standard for developing self-service and enterprise BI solutions
Microsoft Teams automation with Microsoft Graph: bots, APIs, and more
Microsoft Teams: Building on the fastest growing app in Microsoft history
Microsoft's journey to becoming an open source enterprise with GitHub
Migrating your applications, data, and infrastructure to Azure
Moving the web forward with Microsoft Edge
No more last mile problems! Quickly deploy .NET, Python, Java and Node apps on Azure App Service
One Language to Rule Them All: TypeScript
Open Neural Network Exchange (ONNX) in the enterprise: how Microsoft scales ML across the world and across devices
Optimizing your applications for Windows Virtual Desktop
Power BI & Azure Data Services - Better together
Power BI Meets Azure SQL Data Warehouse: Amplifying your data stack with petabyte-scale analytics
Productive front-end development with JavaScript, Visual Studio Code, and Azure
Quickly power your app to handle millions with App Center’s new mobile backend services
Real-time Analytics with Azure Cosmos DB and Apache Spark
Realizing quantum solutions today with Quantum Inspired Optimization and the Microsoft Quantum Development Kit chemistry library
Roadmap for Enterprise Modeling with Power BI and Azure AS
Run AI powered apps on the edge with Azure Data Box Edge
Securely running apps at scale on Azure App Service
Ship it to every platform with Azure Pipelines
Simplifying your app’s user sign-in and authentication using the Microsoft identity platform
Sprinkle some DevOps on your PowerApps & Dynamics 365 Customer Engagement projects
State of the Union: The Windows Presentation Platform
Take the right path: 5 ways to modernize your .NET apps with Windows Server Containers
The Future of C#
The joys and trials of writing cross-platform C++ code – let tools help
The new Windows subsystem for Linux architecture: a deep dive
The Next Transformation in Mobile Development with Xamarin
There is strength in numbers – of resilient application patterns in Azure
Use your Windows developer skills to become an IoT developer - Windows IoT update
Using IoT to improve people’s health and brain power
Visual Studio Code Tips and Tricks – now with… (join us to find out)
Want to *actually* do machine learning? Wrangle data, build models, and deploy them with Azure Machine Learning
Welcome to the world of Machine Learning with ML.NET 1.0
What's new with Azure Resource Manager (ARM)
What’s new in TypeScript
What’s new with Application Insights and Azure Monitor
Windows AI Platform: Fast and practical ML for app developers
Your Brand, Your Assistant - How to build your own voice-first Virtual Assistant